The grid supports various methods of data source binding. One of these methods is binding to data sources that implement IList, IBindingList or IListSource interface via Grid.DataSource property. Objects in the specified collection may be of different types, from simple string[] or object[] data to objects of arbitrary classes, including objects implementing INotifyPropertyChanged interface.
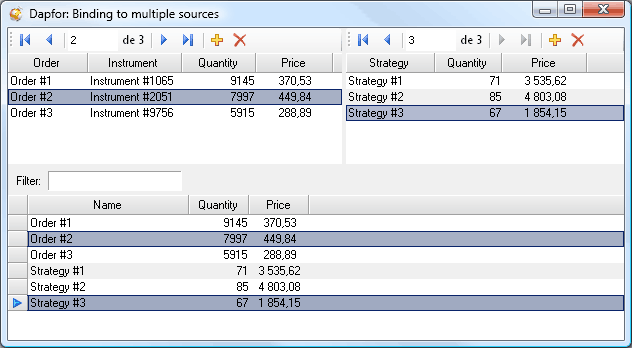
Binding to IList collections enables the grid to create rows at the time of binding. Such collections usually contain a certain number of objects that don’t change during application execution. Use of these collections saves memory used by the application.
Use of IBindingList and its BindingList<T> implementation enables the grid to receive notifications of changes in these collections and to control its content automatically (including data filtering, sorting and grouping operations).
IListSource is used for simultaneous binding to multiple data sources:
![]() | |
---|---|
// Enables to combine multiple data sources (Orders and Baskets) at the same hierarchical level public class MultipleDataSources : IListSource { private readonly IList _sources; public MultipleDataSources(IList[] sources) { _sources = sources; } public IList GetList() { return _sources; } public bool ContainsListCollection { get { return true; } } } //Bind the grid to multiple data sources public void PopulateGrid(Grid grid, IBindingList baskets, IBindingList singleOrders) { grid.DataSource = new MultipleDataSources(new IList[] {baskets, singleOrders}); } |