Application serialization is needed to save and restore user preferences (usually when applications are restarted). If a component cannot save its state and restore it, this task might be very hard for a programmer. Below we provide an example of serializing two grids in an XML file and restoring its state.
.Net Grid has SerializationState property that returns an object implementing ISerializable and IXmlSerializable interfaces. Therefore, this object can be serialized to XML and in binary files. Serialization in the XML format can be done with XmlSerializer class. Let's shows how to serialize and deserialize two grids into a XML file:
C# | ![]() |
---|---|
Grid grid1 = ... Grid grid2 = ... using (MemoryStream ms = new MemoryStream()) using (XmlWriter w = new XmlTextWriter(ms, null)) { XmlSerializer s = new XmlSerializer(typeof(Grid.GridSerializationState)); //Writing to XML w.WriteStartDocument(); //Open the 'TwoGridsExample' tag w.WriteStartElement("TwoGridsExample"); //Serialize the first grid w.WriteStartElement("NetGrid"); w.WriteAttributeString("name", "grid1"); s.Serialize(w, grid1.SerializationState); w.WriteEndElement(); //Serialize the second grid w.WriteStartElement("NetGrid"); w.WriteAttributeString("name", "grid2"); s.Serialize(w, grid2.SerializationState); w.WriteEndElement(); //Close the 'TwoGridsExample' tag w.WriteEndElement(); //End document w.WriteEndDocument(); w.Close(); ms.Flush(); //XML string to be written into the file string xmlString = new UTF8Encoding().GetString(ms.ToArray()); } |
The above code generates the following file:
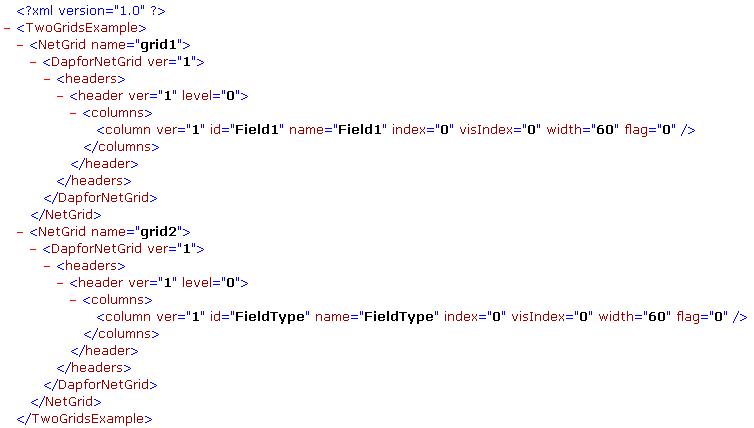
And now we will demonstrate how to deserialize data from the XML file:
C# | ![]() |
---|---|
//1. Create a dictionary to store states Dictionary<string, Grid.GridSerializationState> serializationStates = new Dictionary<string, Grid.GridSerializationState>(); //2. Read from the xml string using (MemoryStream ms = new MemoryStream(new UTF8Encoding().GetBytes(xmlString))) using (XmlReader r = new XmlTextReader(ms)) { XmlSerializer s = new XmlSerializer(typeof(Grid.GridSerializationState)); //Reading from xml r.Read(); //Read from the 'TwoGridsExample' tag while (r.Read()) { r.MoveToElement(); //Read states from 'NetGrid' tags while (r.Read()) { // Move to fist element r.MoveToElement(); string name = r["name"]; string nodeName = r.Name; if (r.Read()) { if (nodeName == "NetGrid") { //Read state serializationStates.Add(name, (Grid.GridSerializationState)s.Deserialize(r)); } r.ReadEndElement(); } } } } //3. The dictionary should have states for each grid grid1.SerializationState = serializationStates["grid1"]; grid2.SerializationState = serializationStates["grid2"]; |
Back to .Net Grid HowTo topics