.Net Grid supports multiple ways of cell editing. When we were developing this mechanism, we based it on standard editors used in the PropertyGrid control.
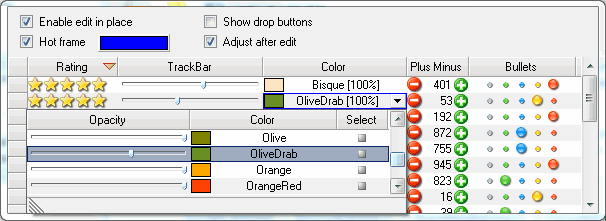
There are several types of these editors including controls that can be displayed in a dropdown box or as a modal dialog. Such editors enable users to edit text, colors and enumerations and to perform painting in small rectangles inside cells. There are plenty of predefined editors. For example, you can get the color editor as follows:
C# | ![]() |
---|---|
UITypeEditor editor = (UITypeEditor)TypeDescriptor.GetEditor(typeof (Color), typeof (UITypeEditor)); |
To edit values, these editors use the EditValue(...) method. Within this method a mandatory control is created and placed in the dropdown box. You should note a very important detail – the return from the function EditValue(...) occurs only when editing within in the control is completed, which is convenient from the programmer's point of view. Look at this example:
C# | ![]() |
---|---|
object EditValue(ITypeDescriptorContext context, IServiceProvider provider, object value) { IWindowsFormsEditorService service = provider.GetService(typeof(IWindowsFormsEditorService)) as IWindowsFormsEditorService; using (SomeControl control = new SomeControl(value)) { service.DropDownControl(control); value = control.NewValue; } return value; } |
The .Net Grid fully supports this mechanism and editors of other vendors that can be used in your applications.
Despite the convenience of this interface, we have concluded that it lacks some functionality. These editors can’t be created above the edited cell with its size (for example, slider control). Besides that, they can’t be painted in the whole cell – just in a small rectangular area. To remediate this, we have created UITypeEditorEx – a class, derived from UITypeEditor that allows to create controls directly above a cell. The process of editing is quite similar to the aforementioned code example:
C# | ![]() |
---|---|
StopEditReason EditCell(IGridEditorService service, Cell cell, StartEditReason reason) { using (SomeControl control = new SomeControl(cell)) { return service.CellEditControl(control, cell.VirtualBounds, reason); } } |
Besides, some editors can be used without creating graphic controls, i.e. a rating editor. It simply draws stars, and when the user clicks on a certain star, this control calculates its relative location and sets a new value. The whole editing code will look as follows:
C# | ![]() |
---|---|
StopEditReason EditCell(IGridEditorService service, Cell cell, StartEditReason reason) { //compute a new rating cell.Value = rating ; return StopEditReason.UserStop ; } |